Hashing is simply passing some data through a formula that produces a result , called a hash.
What is hashing?
Hashing is the process of transforming any given key or string of characters into another value. Most popular use for hashing is the implementation of hash tables.
Hash Function – A hash function is any function that can be used to map data of arbitrary size to fixed-size values. The values returned by a hash function are called hash values, hash codes, digests or simply hashes.
Hash tables – It stores key ad value pairs in a list that is accessible through its index. Key and value pairs are unlimited, the hash function will map the keys to table size.
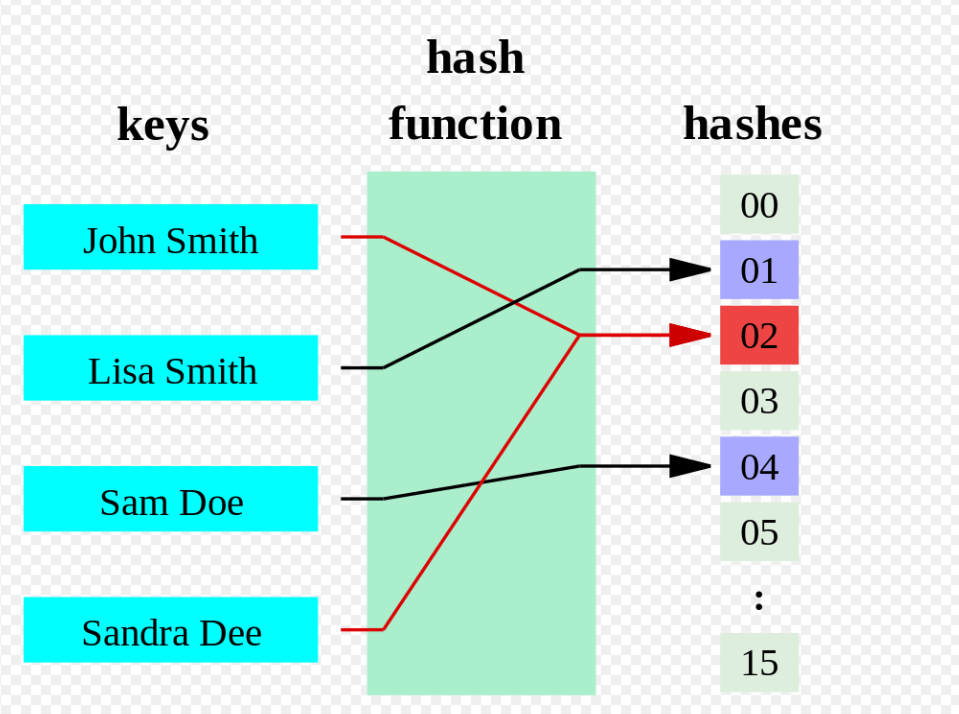
How does hashing works?
Hashing is the process of converting a given key into another smaller value for O(1) retrieval time.
- This is done by taking the help of some function or algorithm which is called as hash function to map data to some encrypted or simplified representative value which is termed as “hash code” or “hash”. This hash is then used as an index to narrow down search criteria to get data quickly.
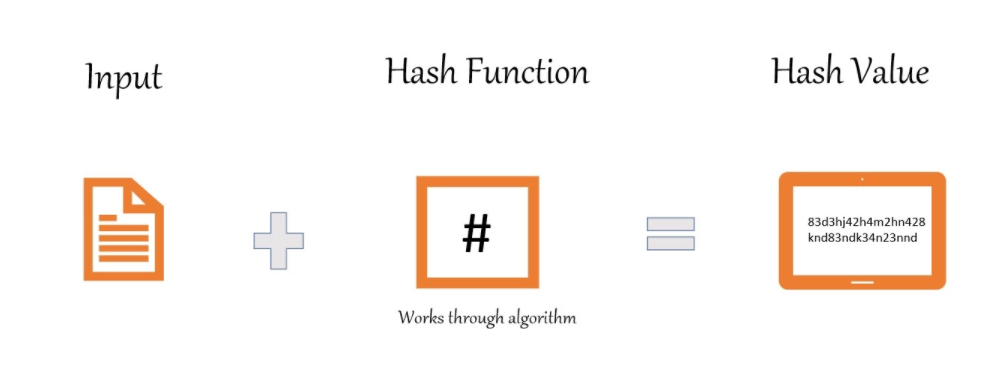
- Hashing can be considered as a significant improvement over DAT to reduce the space complexity.
- In our example of employee system that we have seen in the Introduction part, we can simply pass the employee ID to our hash function, get the hash code and use it as key to query over records.
Applications
Hashing is most commonly used to implement hash tables. A hash table stores key/value pairs in the form of a list where any element can be accessed using its index.
Since there is no limit to the number of key/value pairs, we can use a hash function to map the keys to the size of the table; the hash value becomes the index for a given element.
A simple hashing approach would be to take the modular of the key (assuming it’s numerical) against the table’s size:
index = key \text{ } MOD \text{ } table Size :
index = key MOD table size
This will ensure that the hash is always within the limits of the table size. Here is the code for such a hash function:
def hashModular(key, size):
return key % size
list_ = [None] * 10 # List of size 10
key = 35
index = hashModular(key, len(list_)) # Fit the key into the list size
print("The index for key " + str(key) + " is " + str(index))
Output:
The index for key 35 is 5
Hashing is also used in data encryption. Passwords can be stored in the form of their hashes so that even if a database is breached, plaintext passwords are not accessible. MD5, SHA-1 and SHA-2 are popular cryptographic hashes.
Important technologies in hashing
- Data bucket – Data buckets are memory locations where the records are stored. It is also known as Unit Of Storage.
- Key: A DBMS key is an attribute or set of an attribute which helps you to identify a row(tuple) in a relation(table). This allows you to find the relationship between two tables.
- Hash function: A hash function, is a mapping function which maps all the set of search keys to the address where actual records are placed.
- Linear Probing – Linear probing is a fixed interval between probes. In this method, the next available data block is used to enter the new record, instead of overwriting on the older record.
- Quadratic probing– It helps you to determine the new bucket address. It helps you to add Interval between probes by adding the consecutive output of quadratic polynomial to starting value given by the original computation.
- Hash index – It is an address of the data block. A hash function could be a simple mathematical function to even a complex mathematical function.
- Double Hashing –Double hash is a computer programming method used in hash tables to resolve the issues of has a collision.
- Bucket Overflow: The condition of bucket-overflow is called collision. This is a fatal stage for any static has to function.
Types of hashing
Static : Static hashing is a method of hashing, or shortening a string of characters in computer programming, in which the set of shortened characters remains the same length to improve the ease with which data can be accessed. All objects listed in an object dictionary are static and may not change when static hashing is applied.
Dynamic : Dynamic hashing is a method where the set of shortened characters grows, shrinks and reorganize to fit the way the data is being accessed. All objects listed in an object dictionary are dynamic and may change when dynamic hashing is applied.
https://www.thecyberdelta.com/cyber-attacks-of-2020-21/
https://www.thecyberdelta.com/brute-force/